PBOT2018 with ArduBlocks – Controlling Motors
26 Jun
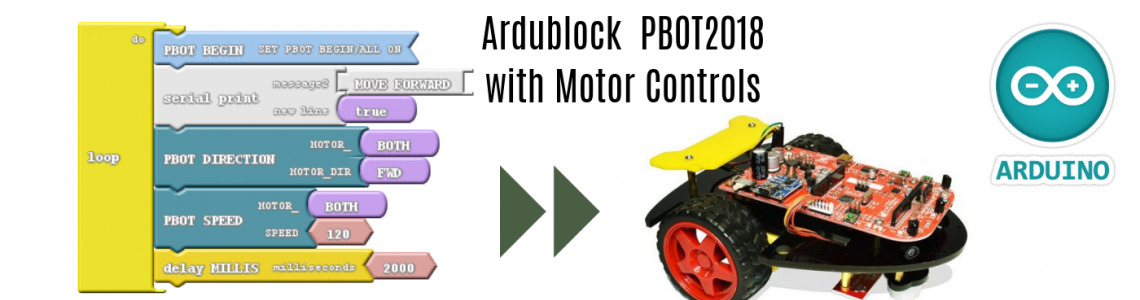
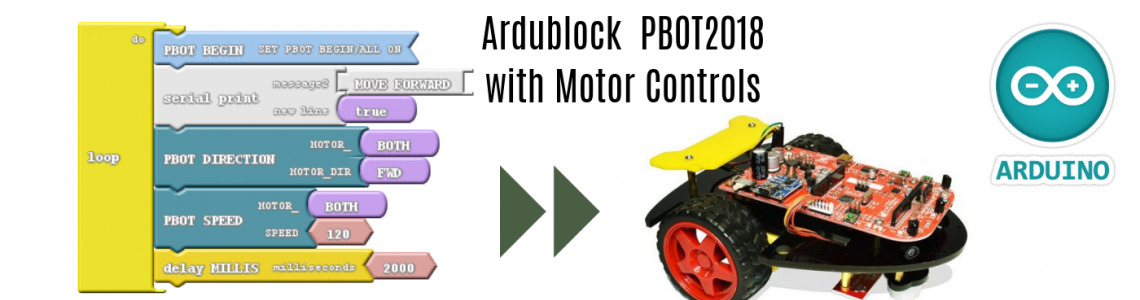
Posted By
0 Comment(s)
1982 View(s)
Updated: 5/17/21
MOTOR DRIVER SECTION:
MOTOR CONNECTIONS:
Left Motor:Blue Wire [1A] - (B), Yellow Wire [1B] - (U) Right Motor: Black Wire [2B] - (B), Green Wire [2A] - (U)
| Top View | Bottom View
- Set Motor Directions:
PBOT.DIRECTION(whichmotor, dir);
- where: whichmotor = MOTOR_A or MOTOR_B, MOTOR_BOTH; dir = MOTOR_FWD, MOTOR_REV.
Ex:
- Set Motor Speeds:
PBOT.SPEED(whichmotor,speed);
- where: whichmotor = MOTOR_A or MOTOR_B, MOTOR_BOTH; speed = 0 to 255
Ex:
GET STARTED:
Setting up your Arduino IDE with ArduBlocks and the eGizmo_PBOT2018 library. (Read Here):
START WITH ADDING A BLOCK "PBOT BEGIN":

SERIAL MONITOR BY ADDING THE SERIAL PRINT BLOCK:

// MOVE FORWARD

// REVERSER MOTORS

Motors reverse at 120 PWM speed in 2secs.
// TURN RIGHT

// TURN LEFT

// MOTOR STOPS

// EXTREME RIGHT
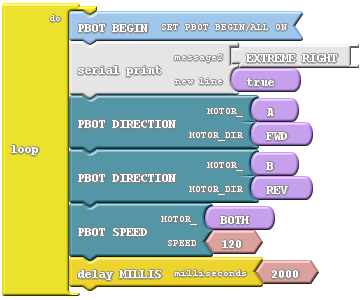
// EXTREME LEFT

- MOTOR_TEST.ino
Leave a Comment